Java Extends Example
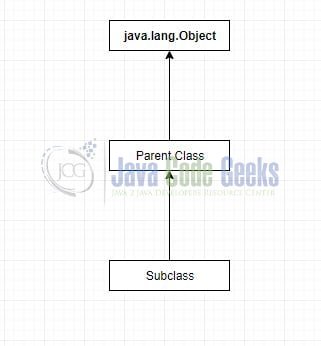
Java Extends Keyword Example Examples Java Code Geeks

What Is Order Of Execution Of Constructors In Java Inheritance

Composition Over Inheritance Wikipedia
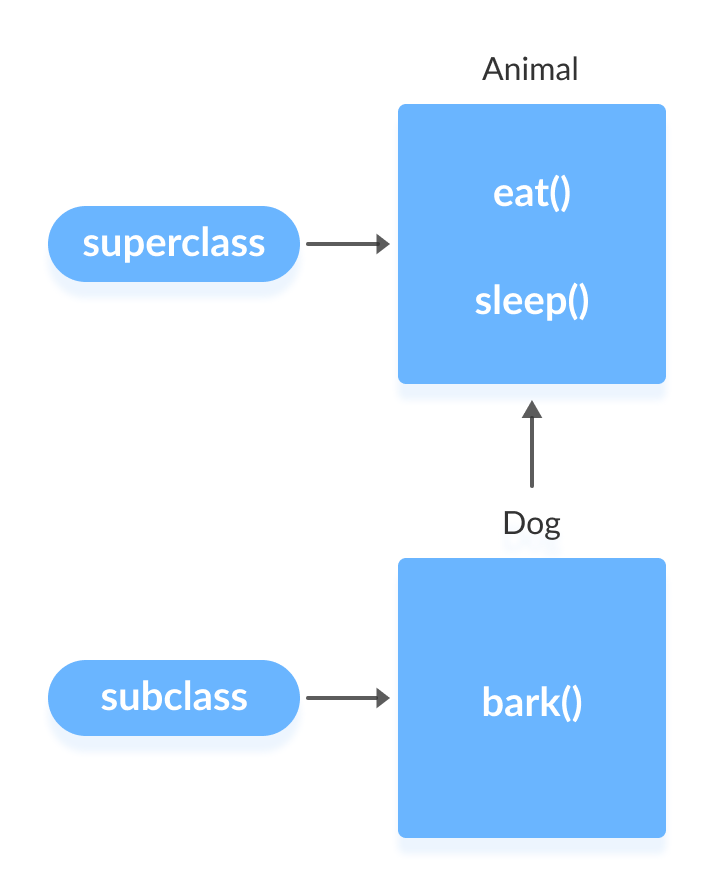
Java Inheritance

Java Interface Extends Example Java Tutorial
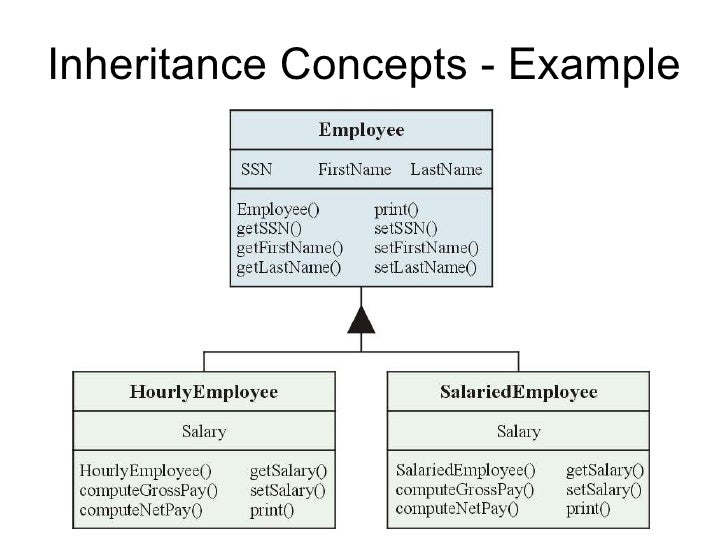
Java Inheritance
A subclass is a class that inherits the functionality of its superclass.
Java extends example. Let’s see an example of this by creating custom checked exception called IncorrectFileNameException:. In the following code, why it doesn't compile, but does when B() is defined?. The Java Platform Class Hierarchy.
We use it while creating a derived class from the parent class or creating a subclass form the superclass. Java is a high level, general purpose programming language developed by James Gosling. // example of extending a class class B { int x = 0;.
So the idea behind this concept is the usability of code, which means when you create a new class (child class). For example, you need an enum to represent basic fonts supported by your system and want third parties to be able to add new fonts. For example, Class X extends class Y to add functionality, either by adding fields or methods to class Y, or by overriding methods of class Y.
To access the interface methods, the interface must be "implemented" (kinda like inherited) by another class with the implements keyword (instead of extends).The body of the interface method is provided by the "implement" class:. JPanel is a Swing’s lightweight container which is used to group a set of components together. For example, the MouseListener interface in the java.awt.event package extended java.util.EventListener, which is defined as − Example.
Following is an example demonstrating Java inheritance. Extending Interfaces in Java Examples by Dinesh Thakur Category:. A class declaration can use the keyword extends on another class, like this:.
An interface is an abstract "class" that is used to group related methods with "empty" bodies:. In this example, we implicitly state that we extend java.lang.Object, and override the functionality of the toString() method by providing our own function. The extends keyword extends a class (indicates that a class is inherited from another class).
In addition, it can also declare new abstract methods and constants. In below example of inheritance, class Bicycle is a base class, class MountainBike is a derived class which extends Bicycle class and class Test is a driver class to run program. Any class that extends Mammal must implement the speak method, and that implementation must have the same signature.
This method will get invoked when a Mouse. Methods of Java MouseListener. In Java, every class is a subclass of java.lang.Object.
Here class XYZ is child class and class ABC is parent class. The concept behind inheritance in Java is that you can create the. It's impossible to specify multiple class names after extends because Java doesn't support class-based multiple inheritance.
The extends keyword indicates that you are making a new class that derives from an existing class. Here, we have inherited the Dog class from the Animal class. Void f1 { x = x+1;} } class C extends B {} public.
This method will get invoked when a Mouse is entering a component.3. Public <T extends Number> List<T> fromArrayToList(T a) {. As you can extend only one class in java, it is not possible to extend any other class if a class extends thread class.You can also implement Runnable interface and then pass it Thread class constructor.
Learn how an interface extends interface in java with example. The main difference between extends and implements in Java is that the keyword “extends” helps to use properties and methods of a superclass while the keyword “implements” allows a class to implement an interface. Java Inheritance is a property of Object-Oriented Programming Concepts by which we can access some methods of a class in another class.
The subclass inherits the fields and methods of the superclass. An interface can inherit or say, can extends another interface or other multiple interfaces in java programs. Note that all classes, whether they state so or not, will be inherit from java.lang.Object.
In this article, we summarize the common practices when working with JPanel in Swing. The class diagram in above is an example of a simple UML diagram, but UML diagrams can get much more complicated. In the example, the Eagle class extends the Bird parent class.
Java code examples to use JPanel in Swing applications. } The keyword extends is used here to mean that the type T extends the upper bound in case of a class or implements an upper bound in case of an interface. Class C extends B { … } When a class C extends class B, C automatically has all variables and methods defined in class B (except private variable and methods).
Note that it doesn’t even come close to explaining all the features of UML. The Animal is the superclass (parent class or base class), and the Dog is a subclass (child class or derived class). When one interface inherits from another interface, that sub-interface inherits all the methods and constants that its super interface declared.
The most common use of extending interfaces occurs when the parent interface does not contain any methods. In this example, we have a base class Teacher and a sub class PhysicsTeacher. In Java, there's the very important keyword extends.
Abstract classes can include abstract methods. In the terminology of Java, a class which is inherited is called a parent or superclass, and the new class is called child or subclass. The solution we came up with was to use an enum and a name-based lookup.
Superclass (parent) - the class being inherited from. In Java, a mixin is an interface which contains a combination of methods from other classes. We will understand them by code example.
Also, example of Interface Extends Multiple Interface in Java program is included. Remember that Java does not allow a class inherits two or more classes directly. In Java, we use the extends keyword to inherit from a class.
HttpServlet is a base class to be extended to create an HTTP servlet suitable for handling HTTP request and providing responses. In short, extends is for extending a class and implements are for implementing an interface. First one is by extending the Thread class and second one is by implementing the Runnable interface.
The child class acquires all the accessible properties from its parent class and can also add some additional properties inside it to provide more information. JPanel is a pretty simple component which, normally, does not have a GUI (except when it is being set an opaque background or has a visual border). When you want to extend a subclass to be extended in inheritance that we use Java extends.
To create a custom checked exception, extends java.lang.Exception. Inheritance allows us to extend a class with child classes that inherit the fields and methods of the parent class. A quick tutorial on how to use extends in java.
Copy and paste the following program in a file with name My_Calculation.java. Difference Between Java Implements and Extends. To create a sub class (child) from a Java super class (parent), the keyword extends is used.
You then follow the "extends" keyword with the parent class you want to extend. – java 7 for Absolute Beginners. There are two ways to create a thread in java.
This class does not override any of the implementations from the AbstractCollection class, but merely adds implementations for equals() and hashCode() method. Multiple inheritance of state is not allowed:. It’s also called as Java extends the class.
Previous Next In this post, we will see Java Runnable example. Class derived-class extends base-class { //methods and fields } Example:. In Java, we need to use the extends keyword to create a child class.
Interface extends interface in java. It is used to build various applications such as standalone, mobile, web, etc. The extends is a Java keyword, which is used in inheritance process of Java.
The Object class, defined in the java.lang package, defines and implements behavior common to all classes—including the ones that you write. The class XYZ is inheriting the properties and methods of ABC class. Extending a Class that has Explicit Constructors.
The basic element in a class diagram is a class. When an implement an interface, we use the keyword implement. What is extends in Java?.
The topics discussed in this article are:. As Java doesn’t support multiple. It is a keyword that indicates the parent class that a subclass is inheriting from and may not.
The keywords extends and implements, both are used to execute the Inheritance concept of Object-Oriented programming, yet, there is a subtle difference between them.This article on extends vs implements in Java will help you understand the major differences between these keywords. Class XYZ extends ABC { } Inheritance Example. All Inheritance in Java with example programs PDF are in Java 11, so it.
The AbstractSet class in Java is a part of the Java Collection Framework which implements the Collection interface and extends the AbstractCollection class.It provides a skeletal implementation of the Set interface. Extending thread class Implementing Runnable interface. In this example, you can observe two classes namely Calculation and My_Calculation.
This extends keyword establishes the inheritance relationship between two classes. In the Java platform, many classes derive directly from Object, other classes derive from some of those classes, and so on, forming a hierarchy of classes. Public interface MouseListener extends EventListener.
Extending java.lang.Enum All enums implicitly extend java.lang.Enum. Both will be done by implementing a mixin interface. The StudentResults class will be the super class and the Certificates class will be the sub class.
For example, our Mammal class includes an abstract speak() method. There are five methods. Example of Java extends keyword Let’s see an example that uses the extends keyword in Java.
It specifies the super class in a class declaration using extends keyword. Take a look at the following example, which demonstrates the use of the 'extends' keyword. We usually override methods like doGet(), doPost() etc.
Public class ClassA { public ClassA() { //this is the constructor of ClassA. The extends keyword in Java is useful when we want to inherit the properties and methods of a parent class in our child class. Example public interface Hockey extends Sports, Event ging Interfaces.
Our next example shows a more common use of the keyword 'extends'. Using extends keyword, the My_Calculation inherits the methods addition () and Subtraction () of Calculation class. Java extends example Here is an example of how to extends a class in java.
This class extends another class (Parent class) and reuse methods, variables, and fields of the parent class. In Java, it is possible to inherit attributes and methods from one class to another. To extend an interface, you use the extends keyword.
Any class that extends a class with an abstract method must implement that method. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
Subclass (child) - the class that inherits from another class;. We group the "inheritance concept" into two categories:. The class whose methods is inherited know as Parent class/ Base class/ Superclass, and the class which is derived from Parent class is known as Child class/Subclass.
These examples codify is-a relationships:. Public class IncorrectFileNameException extends Exception { public IncorrectFileNameException(String errorMessage) {. Public class MyFrame extends JFrame implements ActionListener { } 6.
The java extends keyword is used to define that a class is a subclass. In practice with Swing development, it’s common to have a class extended a JFrame and implements an event listener interface, for example:. The following information describe the details of creating UML class diagrams.
The subtyping relationship is preserved as long as we don’t change the type argument, below shows an example of multiple type parameters. To inherit a class we use extends keyword. Java Servlet - Extending HttpServlet Examples:.
This method will get invoked when a Mouse button is clicked on a component.2. We want to create a sub class from the StudentResults class. Let's see the examples of creating a thread.
To create a custom exception, we have to extend the java.lang.Exception class. It’s an excellent way to achieve code reusability. Car is a specialized Vehicle.
Consider the example below. As you might know, there are two ways of creating threads in java. The meaning of "extends" is to increase the functionality.
In Java, a class can only extend one parent and therefore an enum cannot extend any other class (but implement interfaces. Inheritance is widely used in java applications, for example extending Exception class to create an application specific Exception class that contains more information like error codes. Java Servlet JAVA EE.
The Java Tutorials have been written for JDK 8. For example, ArrayList<E> implements List<E> that extends Collection<E>, so ArrayList<String> is a subtype of List<String> and List<String> is subtype of Collection<String>.
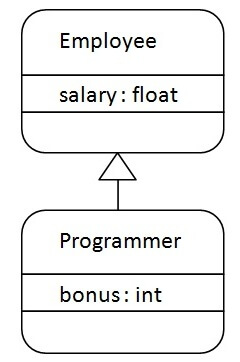
Inheritance In Java Javatpoint
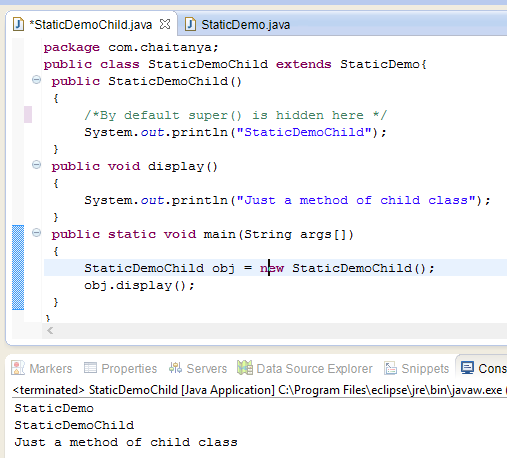
Java Static Constructor Is It Really Possible To Have Them In Java
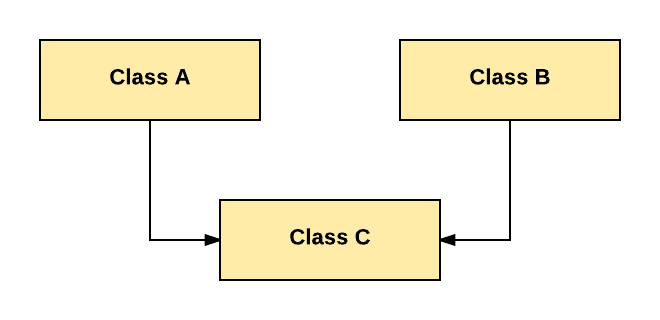
Inheritance In Java Oops With Example

The Intricacies Of Multiple Inheritance In Java
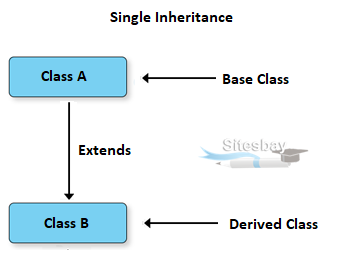
Inheritance In Java Real Life Example Of Inheritance In Java
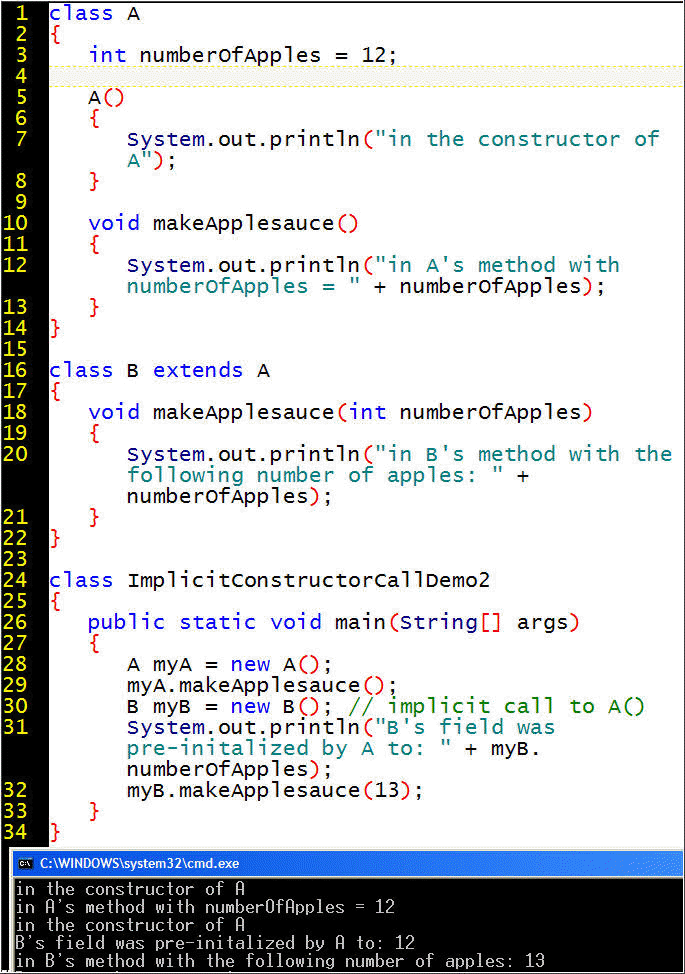
First Course In Java Session 7
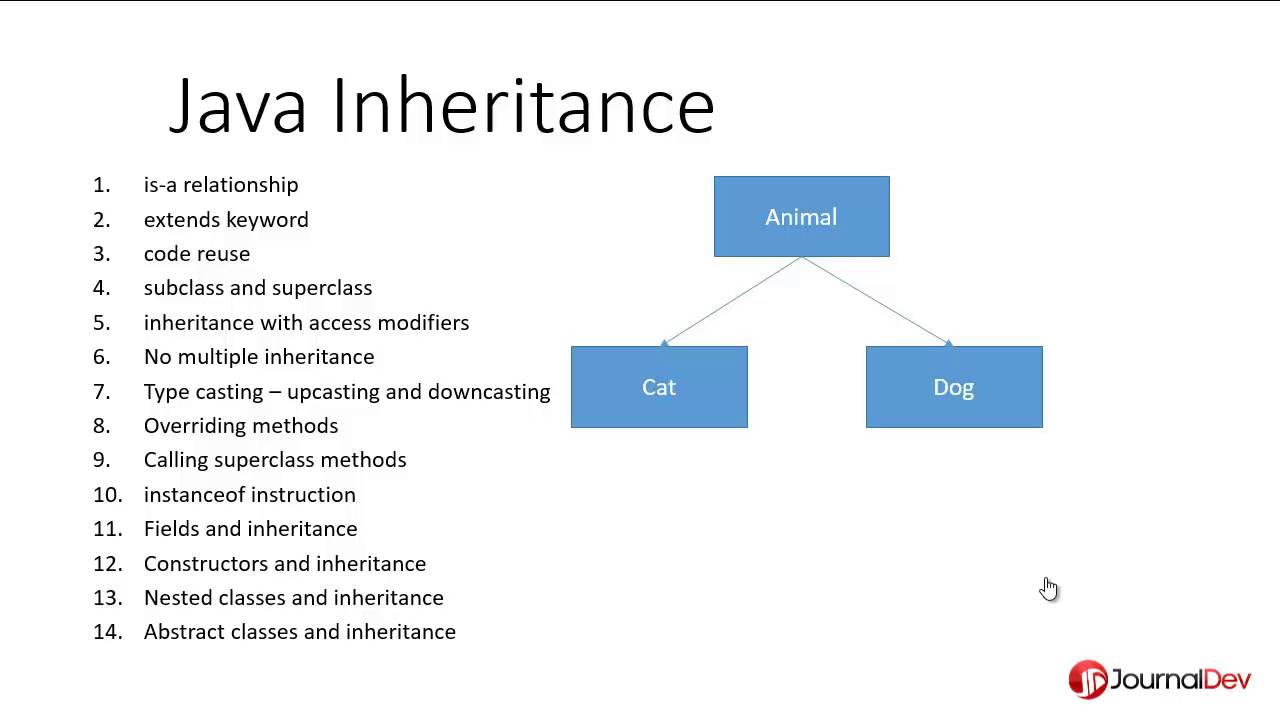
Inheritance In Java Java Inheritance Tutorial Part 2 Youtube
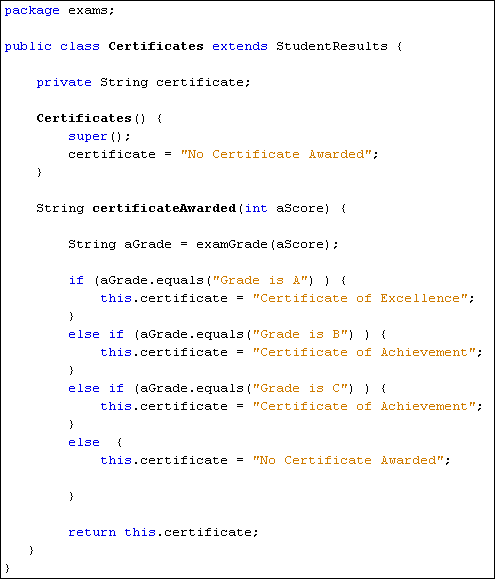
Java For Complete Beginners Inheritance
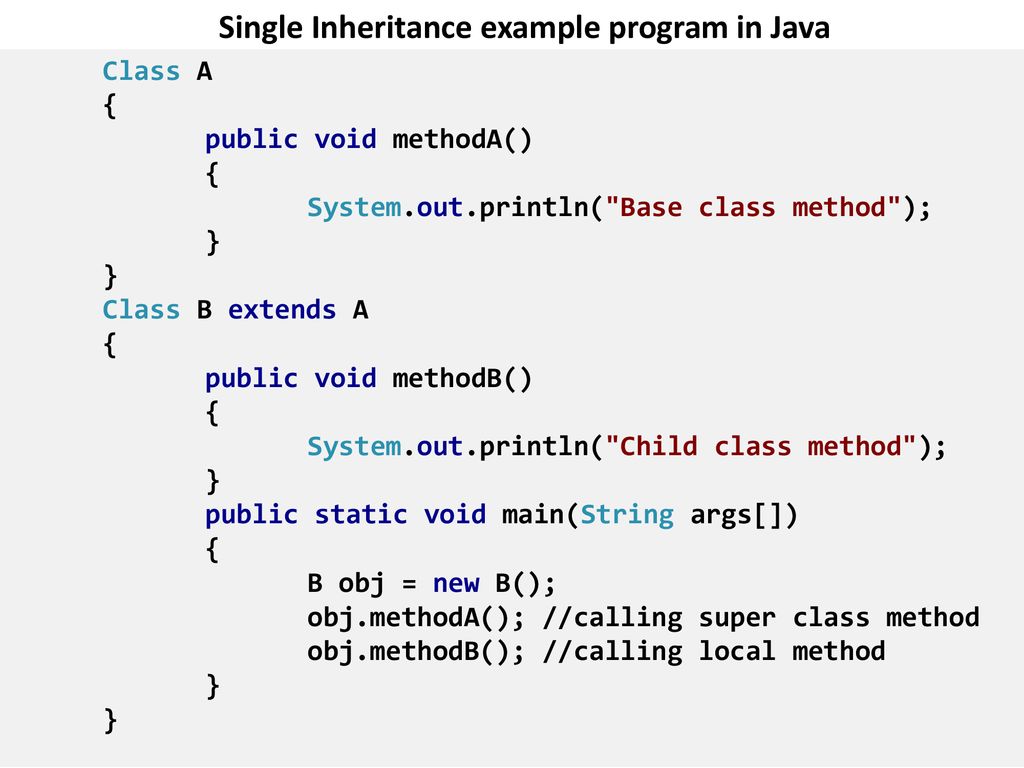
Inheritance In Java Ppt Download
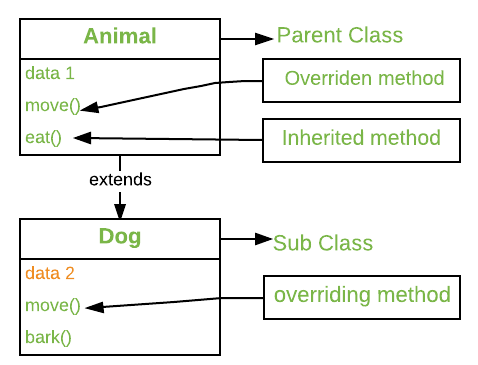
Overriding In Java Geeksforgeeks
1

What Is Inheritance In Java Bytesofgigabytes
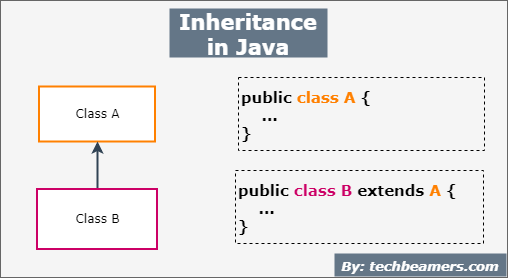
Java Inheritance For Beginners Explained With Examples
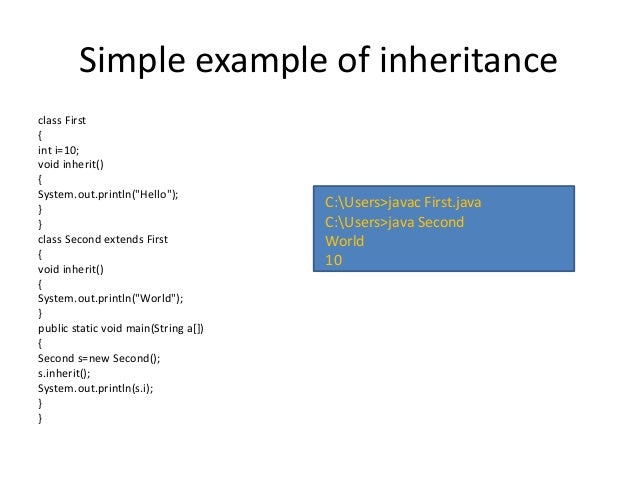
Multiple Inheritance Possible In Java

Why Favor Composition Over Inheritance Java Oop Interview Q As

Java Tutorials Inheritance Basics
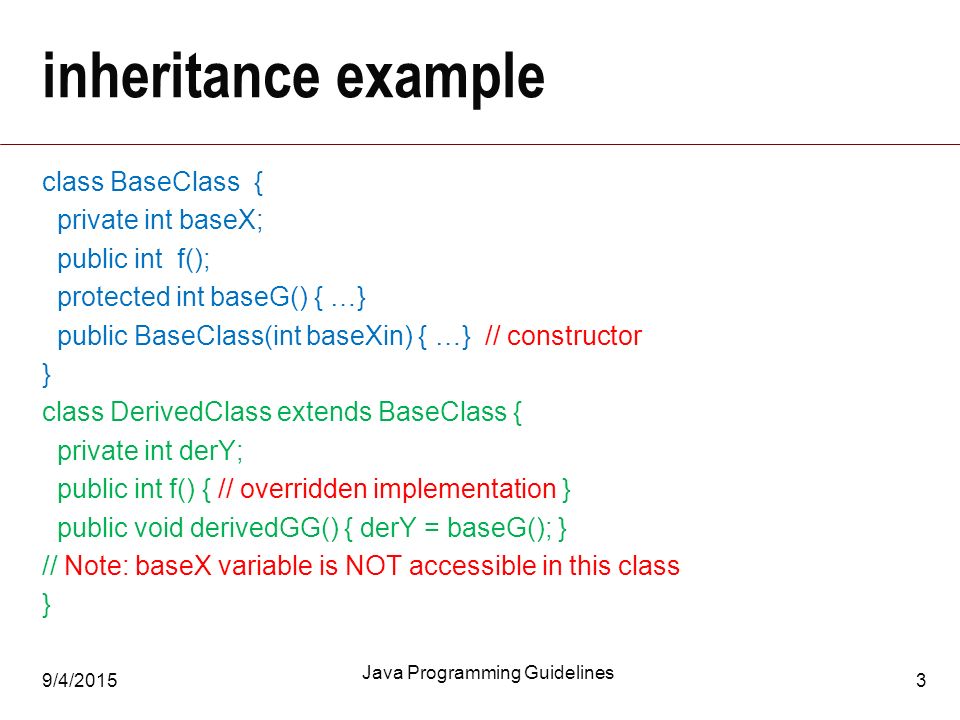
Inheritance Using Java Ppt Video Online Download
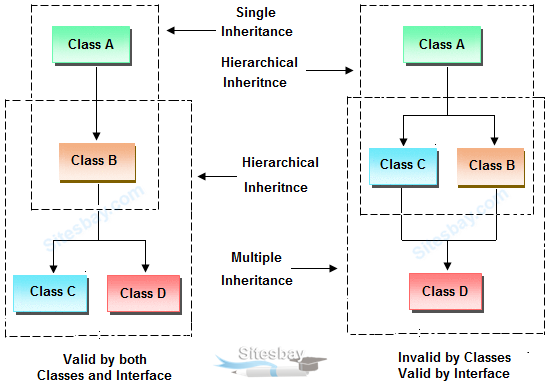
Inheritance In Java Real Life Example Of Inheritance In Java
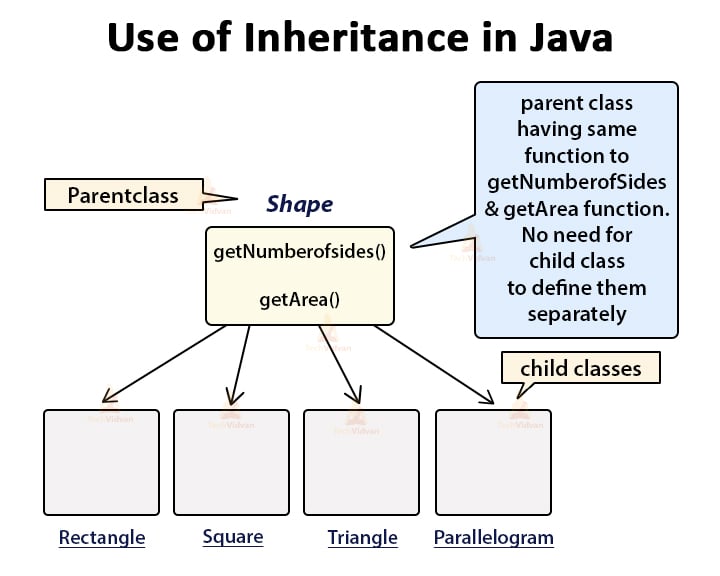
Java Inheritance Types Importance Of Inheritance With Real Life Examples Techvidvan
Java Persistence Inheritance Wikibooks Open Books For An Open World

Inheritance In Java
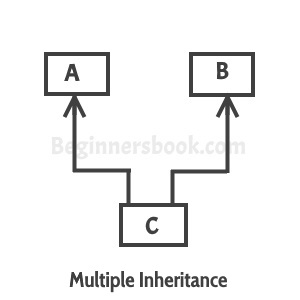
Inheritance In Java Programming With Examples
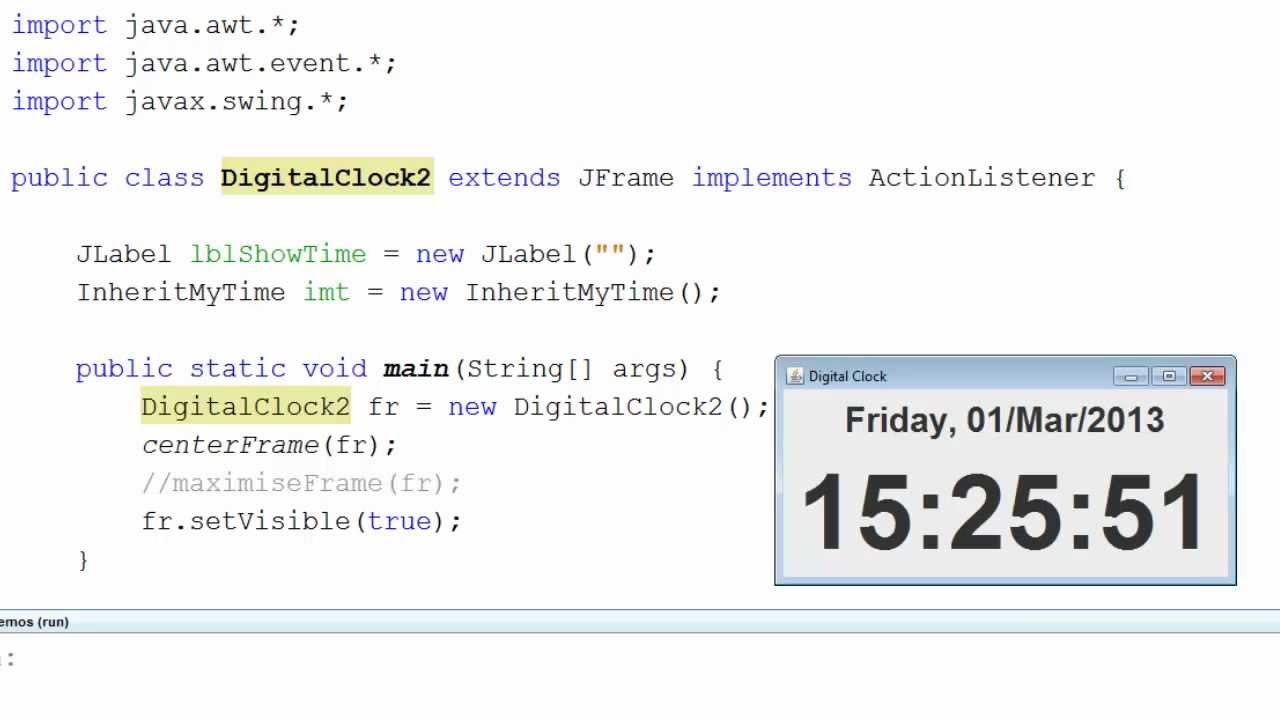
Java Multiple Inheritance Youtube
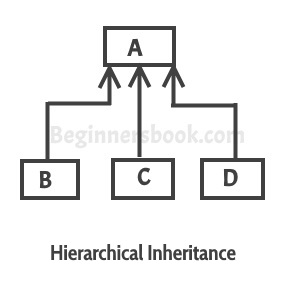
Inheritance In Java Programming With Examples

Inheritance In Java Core Java Tutorial Minigranth

Java Inheritance Example Vehicle Java Tutorial Youtube
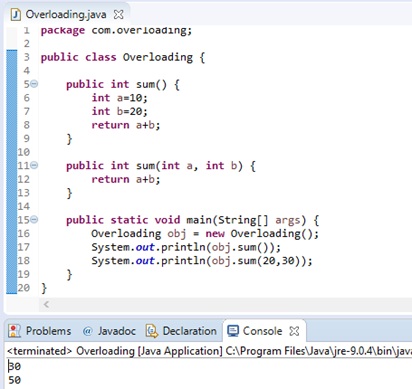
What Is The Difference Between Inheritance And Polymorphism In Java Pediaa Com

Hierarchical Inheritance In Java
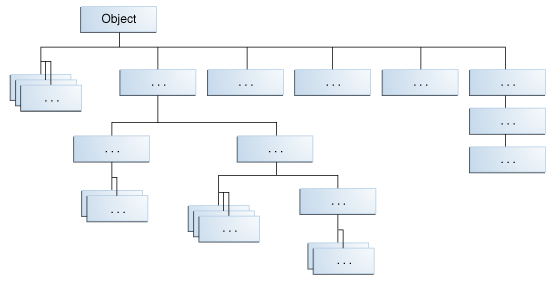
Inheritance The Java Tutorials Learning The Java Language Interfaces And Inheritance

Inheritance And Its Type In Java
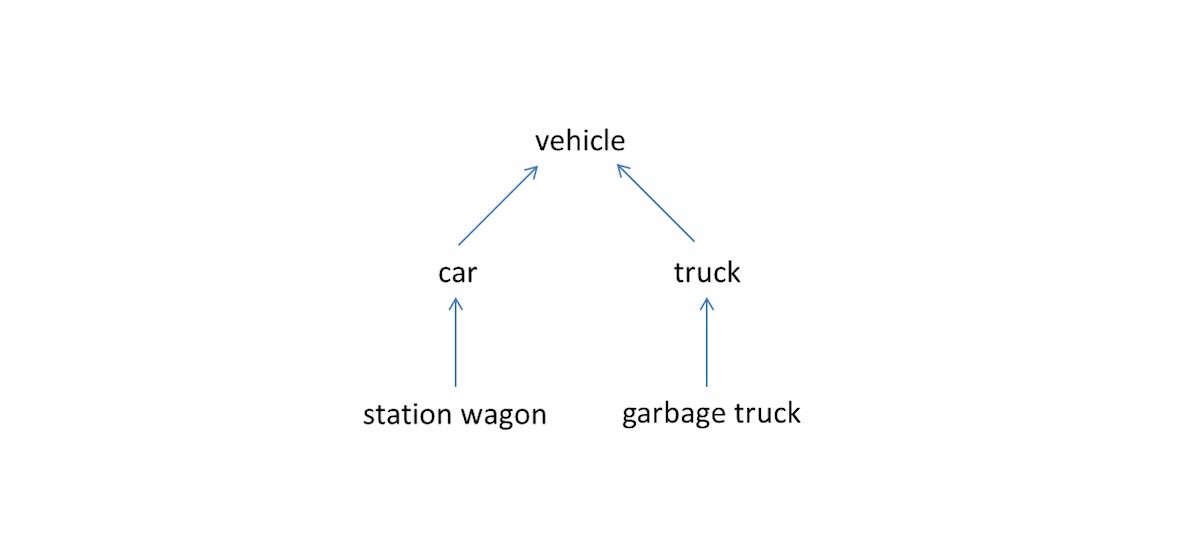
Inheritance In Java Part 1 The Extends Keyword Infoworld

Inheritance In Java Types Of Inheritance Simple Snippets

Inheritance In Java Core Java Tutorial Minigranth

Java Saurabhjain1537
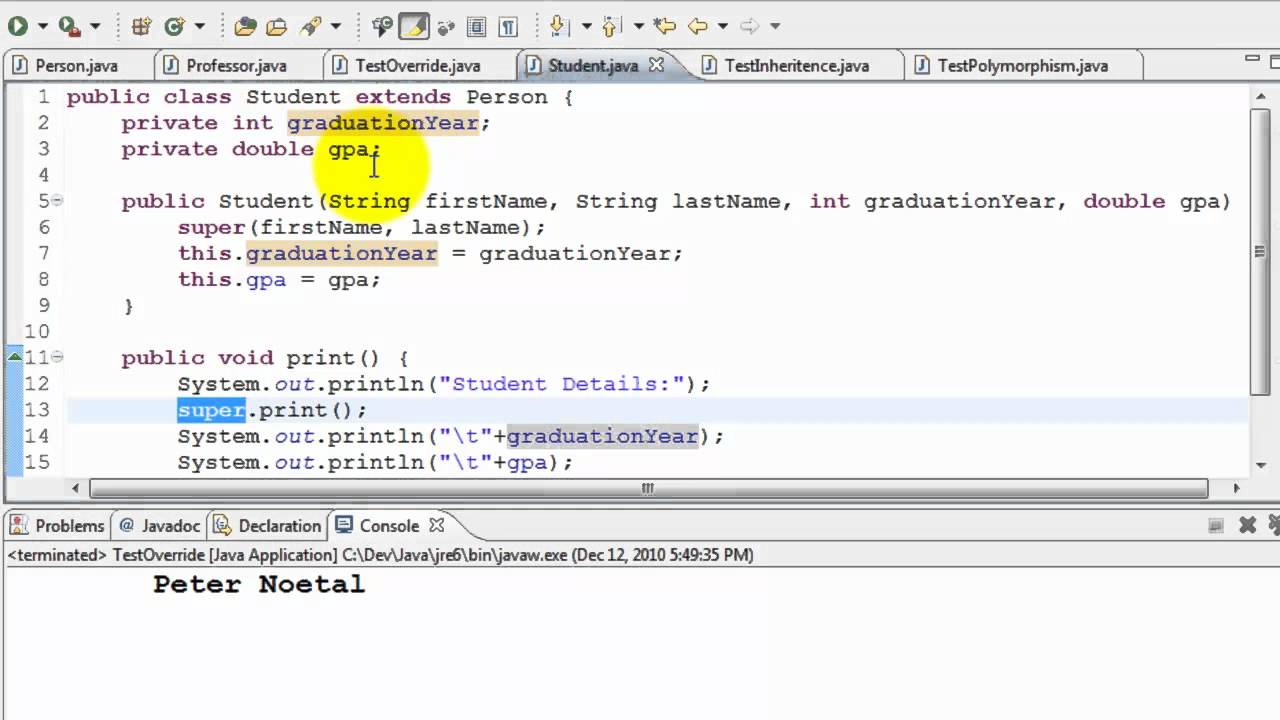
Java Tutorial Inheritance And Polymorphism Youtube

Inheritance In Java Coding Guru
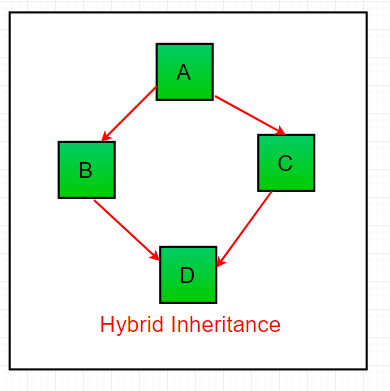
Inheritance In Java Geeksforgeeks
Q Tbn 3aand9gcqrtf5qse0yrmxawch7ckq Hsynlrgnlikkzx5rvlgkjabr0x8a Usqp Cau

Inheritance In Java Coding Guru
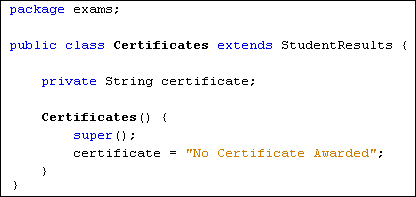
Java For Complete Beginners Inheritance

Interfaces Can Be Extended Java A Beginner S Guide 5th Edition 5th Edition Book

Inheritance In Java Inheritance In Java Is Simply That When By Talha Bhurgri Talha Bhurgri Medium
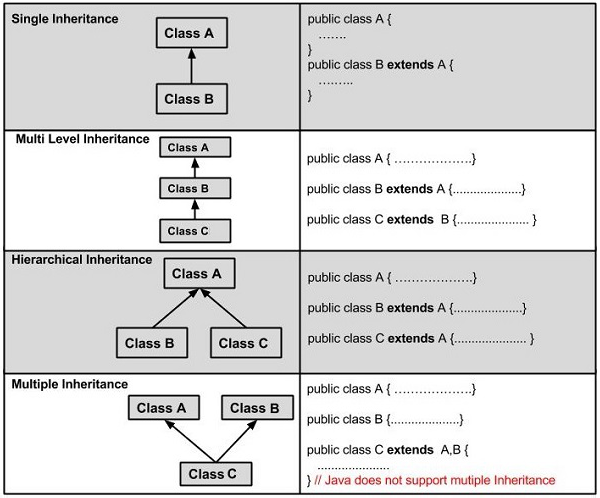
Java Inheritance Tutorialspoint

Java For Complete Beginners Inheritance

Jpa Single Table Inheritance Example Thejavageek

Java Tutorials Inheritance Basics
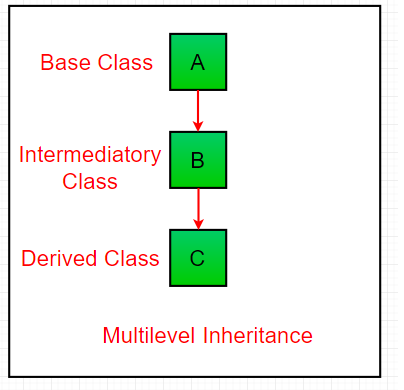
Inheritance In Java Geeksforgeeks

Inheritance In Java Javatpoint
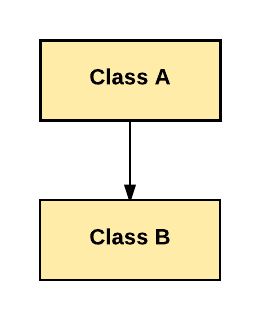
Inheritance In Java Oops With Example
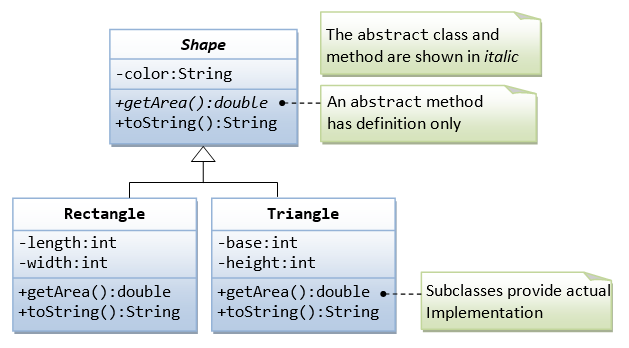
Oop Inheritance Polymorphism Java Programming Tutorial

Java Inheritance Tutorialspoint
Multiple Inheritance Java Bytesofgigabytes

Java Extends Keyword With Examples Techvidvan

Inheritance Vs Polymorphism In Java Study Com
Q Tbn 3aand9gcrh7us5hmwmolowosov0 V X0xzswq9x6tfzqvxbg Jkrsomb5w Usqp Cau

Extending Java Classes Using Proxy Getting Clojure

Inheritance In Java Programming Language With Examples

Polymorphism In Java Inheritance In Java

Java Method Overriding Examples And Concepts Overriding Rules Crunchify

Java Inheritance Example Inheritance In Java

Jpa Joined Inheritance Example Thejavageek
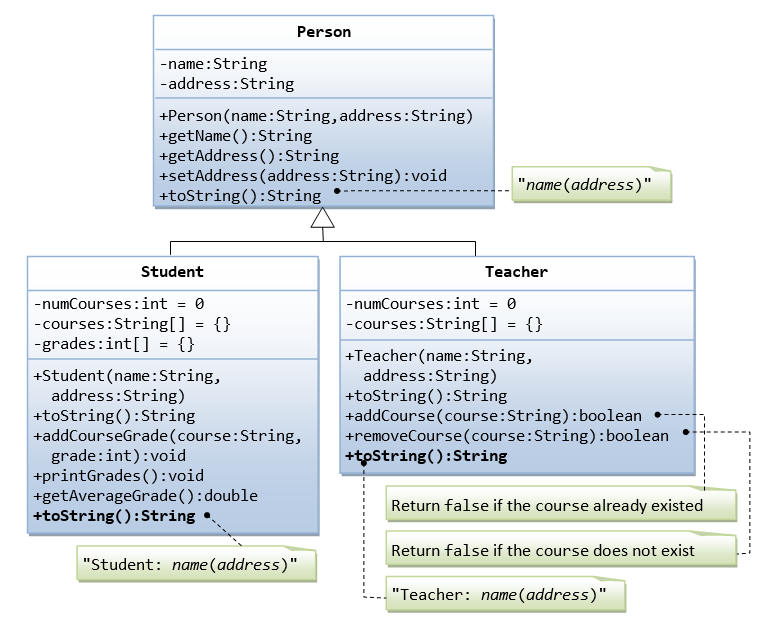
Oop Inheritance Polymorphism Java Programming Tutorial

Java Composition Inheritance Delegation Examples Bestprog
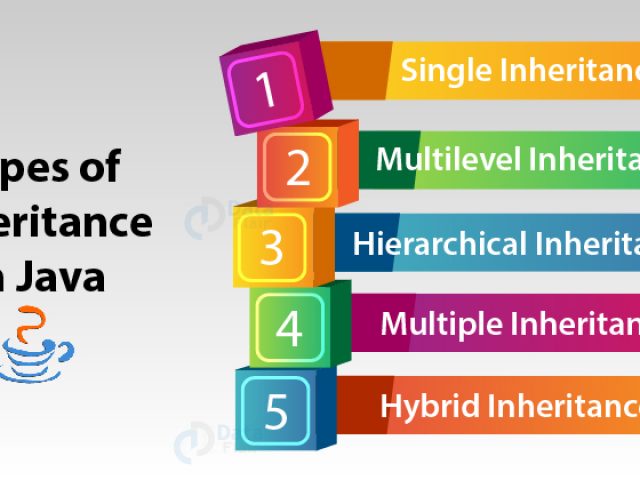
Inheritance In Java Types With Example You Can T Afford To Miss Out Dataflair
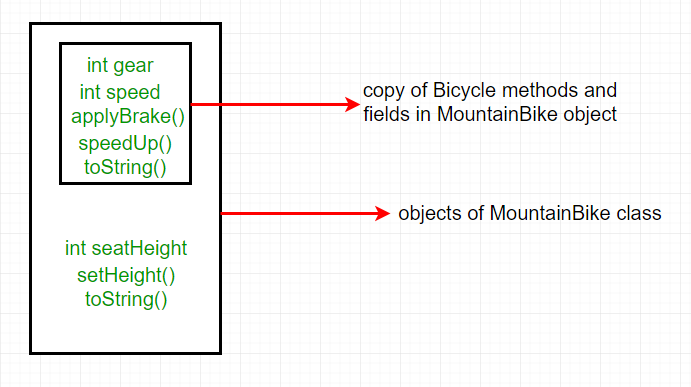
Inheritance In Java Geeksforgeeks
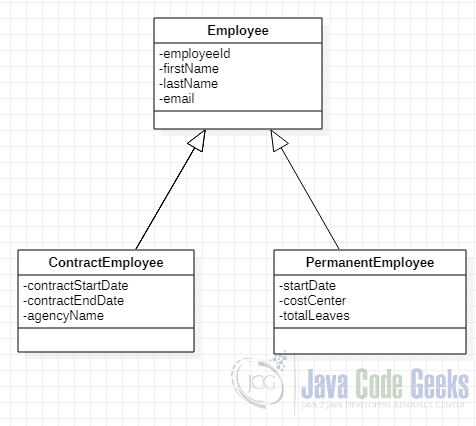
Hibernate Inheritance Mapping Examples Java Code Geeks
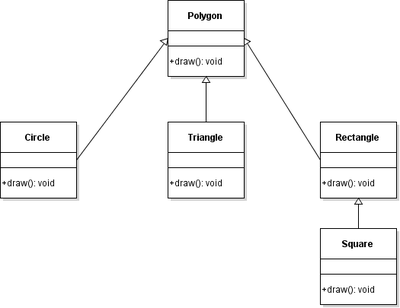
Inheritance In Java Dariawan

Java Extends Class Interface Keyword Multiple Class Example Eyehunts
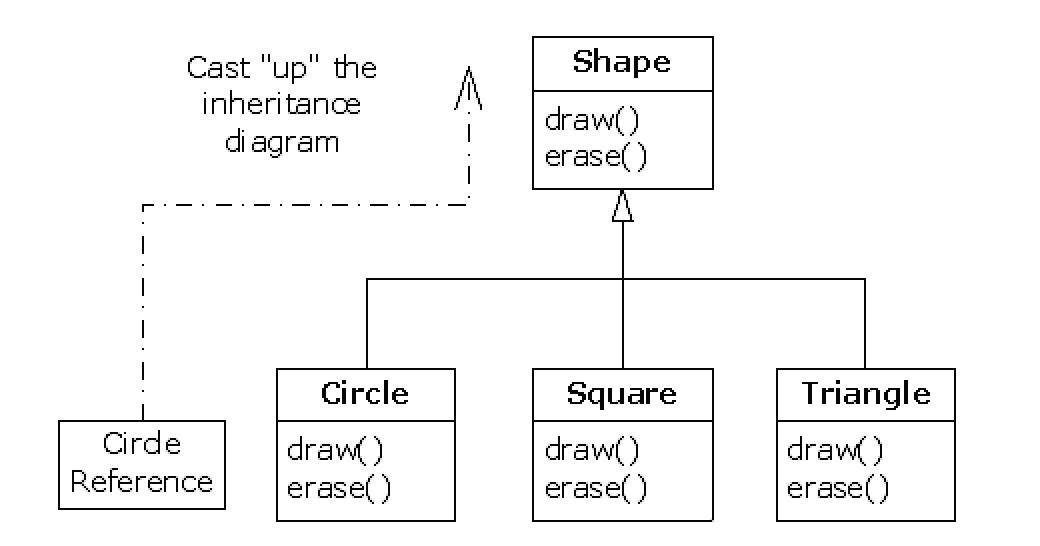
Top 21 Java Inheritance Interview Questions And Answers Java67
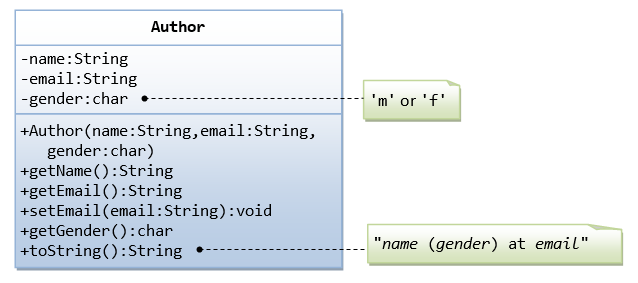
Oop Inheritance Polymorphism Java Programming Tutorial

Java Inheritance Is A Facing Issues On It

Inheritance And Its Type In Java

Practical Application For Java Using Inheritance Study Com
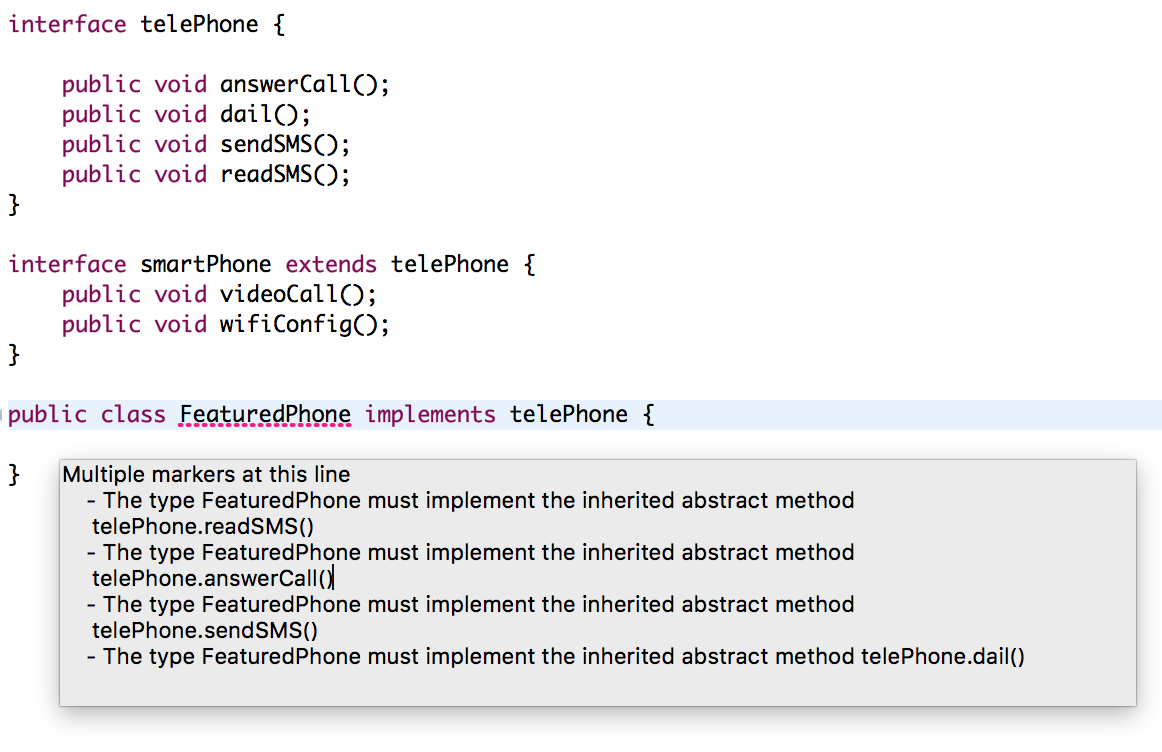
Java Discover Interface Extends Another Interface In Java

How To Code Inheritance In Java Beginner S Tutorial In Oop By Rishi Sidhu Towards Data Science

Inheritance And Polymorphism

What Is Inheritance In Java Quora
Extends Java Keyword With Examples

Java Tutorial Inheritance Example Codes I M Rubel Java Tutorial Technology Lessons Java
1
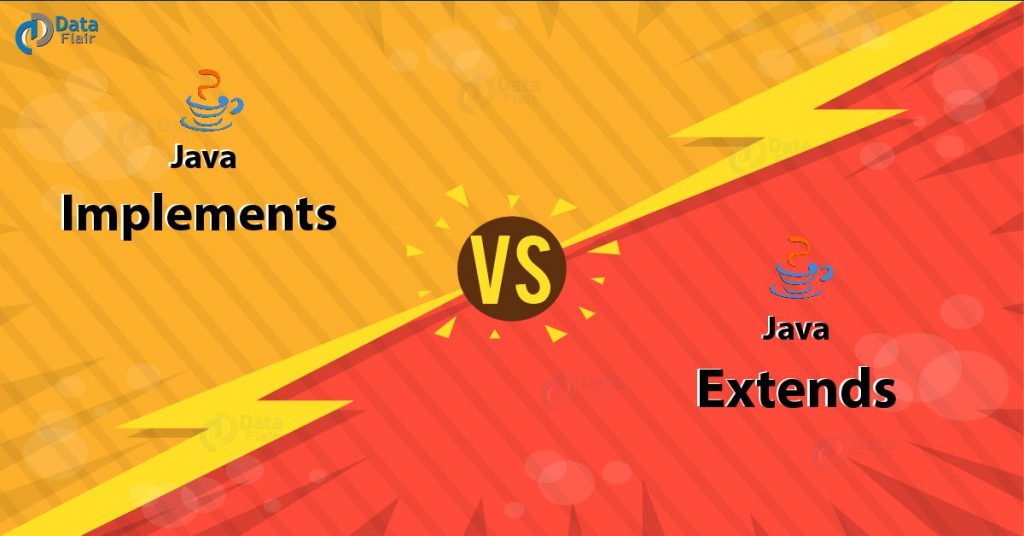
Java Extends Vs Implements With Example Program Dataflair
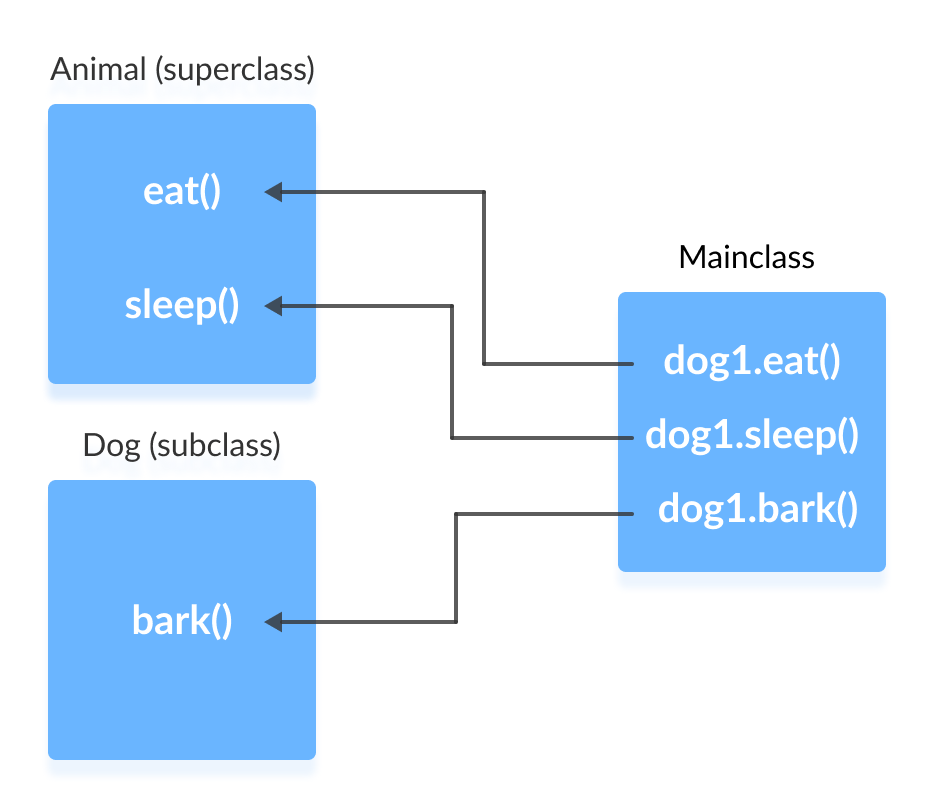
Java Inheritance
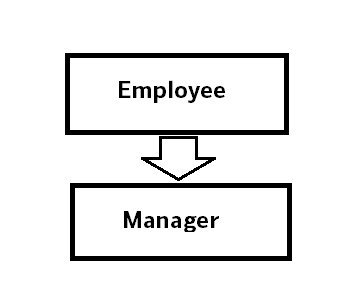
Oop Inheritance Types Of Inheritance Howtodoinjava
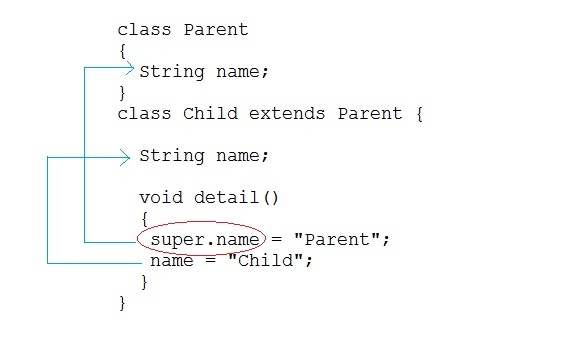
Inheritance In Java Core Java Tutorial Studytonight

Inheritance In Java Core Java Tutorial Minigranth

Inheritance In Java Example Journaldev
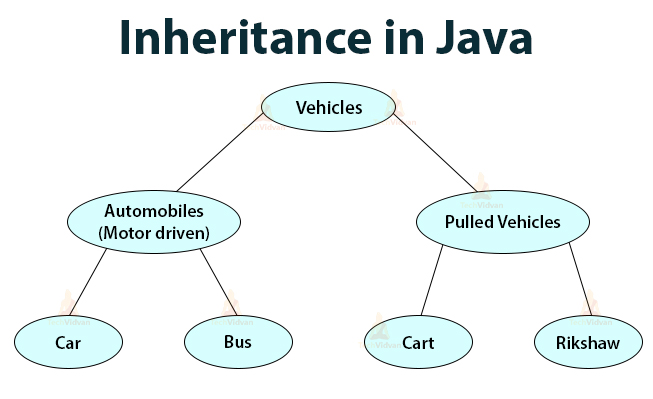
Java Inheritance Types Importance Of Inheritance With Real Life Examples Techvidvan

Java Timer And Timertask Reminder Class Tutorials Example Crunchify
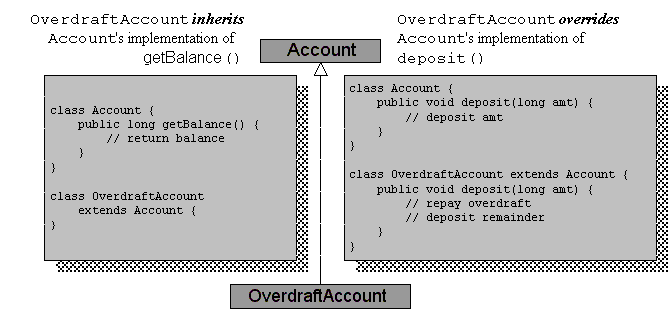
Objects And Java Seminar Composition And Inheritance
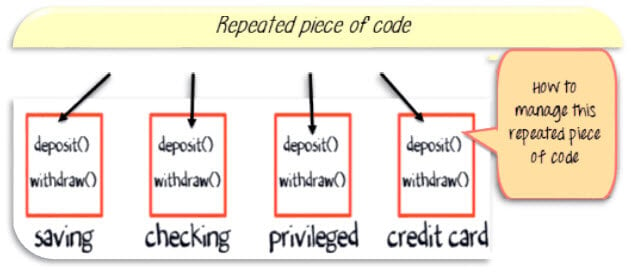
Inheritance In Java Oops With Example
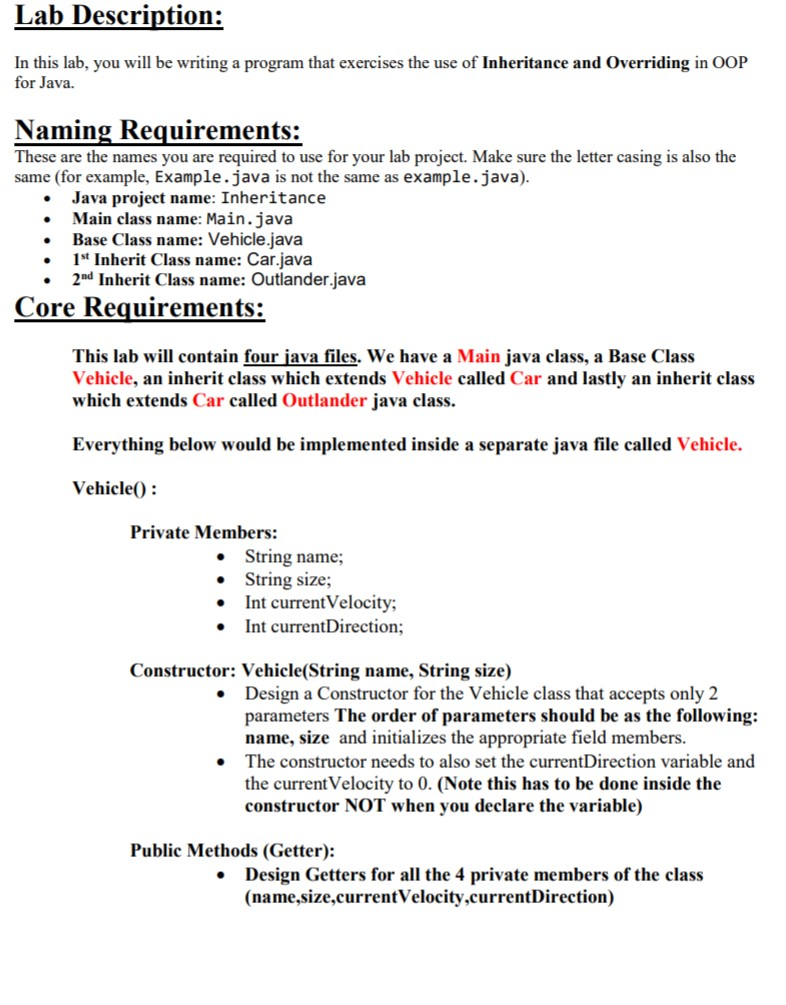
Solved Lab Description In This Lab You Will Be Writing Chegg Com
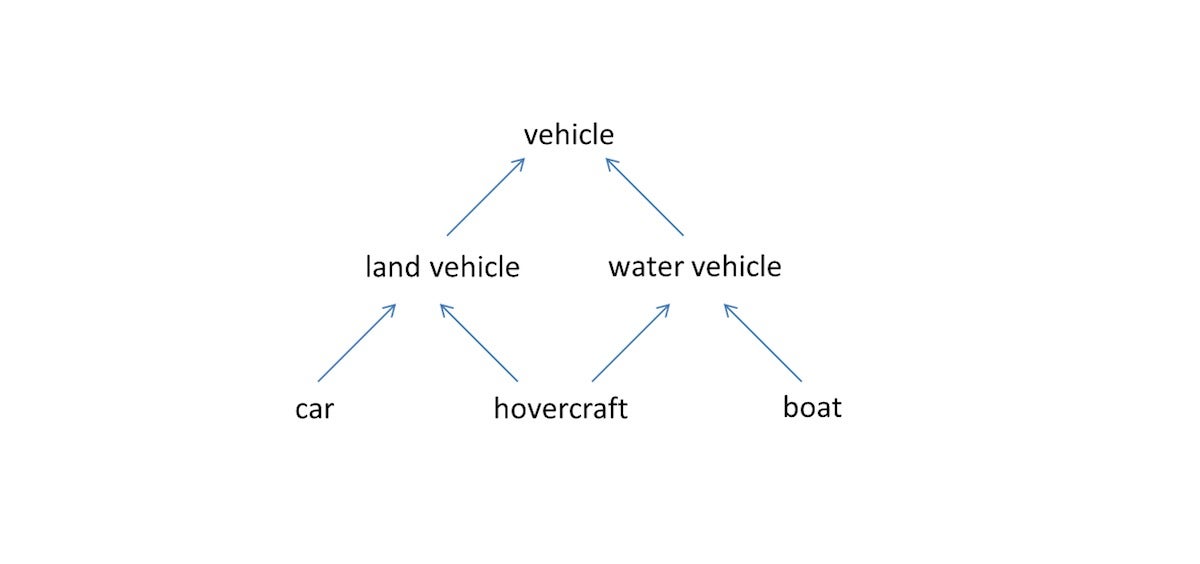
Inheritance In Java Part 1 The Extends Keyword Infoworld

What Is The Difference Between Java Subtype And True Subtype Stack Overflow

Types Of Inheritance In Java And Oops With Code Example And Diagram
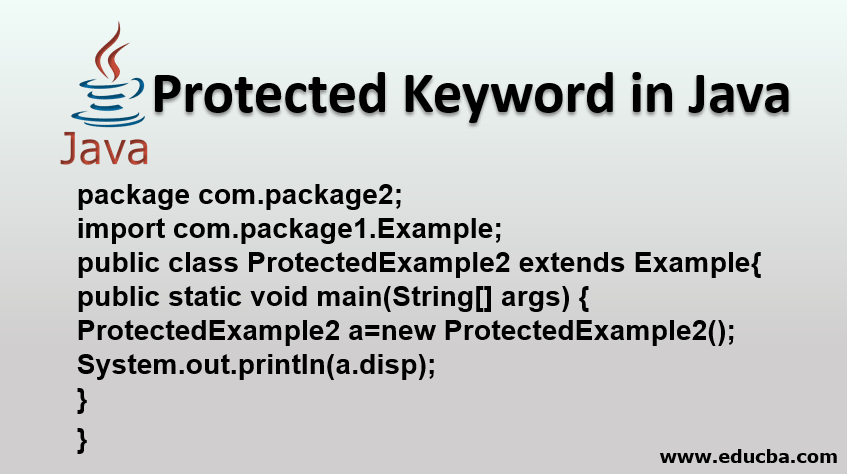
Protected Keyword In Java Uses Of Protected Keyword In Java

Multiple Inheritance In Java Java Tutorials Dream In Code

Multilevel Inheritance In Java
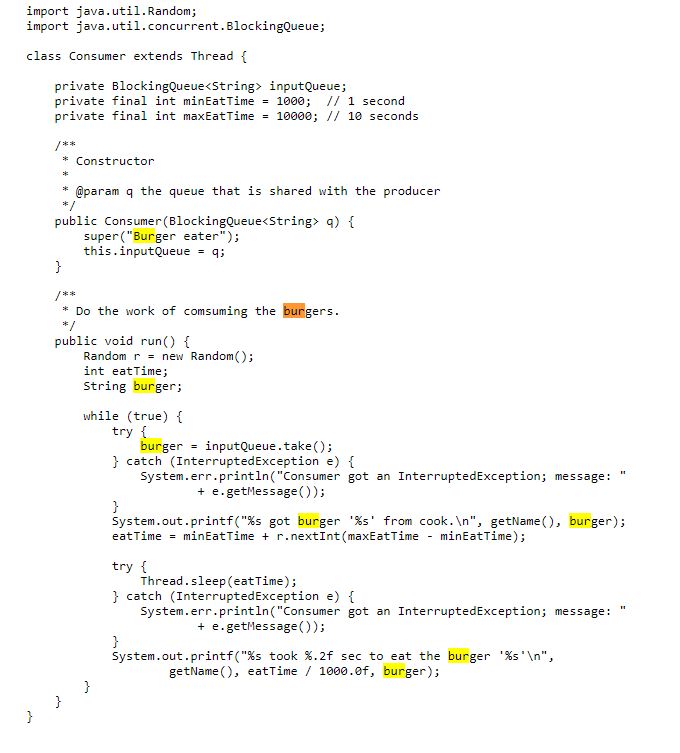
Need Answer Written In Java Thanks Extend The Bur Chegg Com